[Android开发练习3] 四季图的切换
阿里云国内75折 回扣 微信号:monov8 |
阿里云国际,腾讯云国际,低至75折。AWS 93折 免费开户实名账号 代冲值 优惠多多 微信号:monov8 飞机:@monov6 |
前言
本题主要涉及到使用帧式布局以及如何为组件设置点击的事件响应包括获取事件源设置事件监听器在Activity文件中书写一般的业务逻辑代码。
文章目录
布局代码
text3_season.xmllayout
使用帧式布局FrameLayout来存放图片控件使用线性布局LinearLayout来存放四个按钮标签全局采用相对布局RelativeLayout,形成最终的展示页面。四季图可以自行从网上选择下载并将其复制粘贴到drawable文件夹下进行调用。
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<!-- 帧式布局放图片控件-->
<FrameLayout
android:id="@+id/frameSeason"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:tag="春天"
android:src="@drawable/spring"
android:scaleType="fitXY"
android:layout_width="match_parent"
android:layout_height="match_parent"
>
</ImageView>
<ImageView
android:tag="夏天"
android:visibility="gone"
android:src="@drawable/summer"
android:scaleType="fitXY"
android:layout_width="match_parent"
android:layout_height="match_parent"
>
</ImageView>
<ImageView
android:tag="秋天"
android:visibility="gone"
android:src="@drawable/autom"
android:scaleType="fitXY"
android:layout_width="match_parent"
android:layout_height="match_parent"
>
</ImageView>
<ImageView
android:tag="冬天"
android:visibility="gone"
android:src="@drawable/winter"
android:scaleType="fitXY"
android:layout_width="match_parent"
android:layout_height="match_parent"
>
</ImageView>
</FrameLayout>
<!-- 线性布局放标签按钮-->
<LinearLayout
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:layout_marginBottom="30dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<TextView
android:id="@+id/spring"
android:text="春天"
style="@style/season_name"
>
</TextView>
<TextView
android:id="@+id/summer"
android:text="夏天"
style="@style/season_name"
>
</TextView>
<TextView
android:id="@+id/autuom"
android:text="秋天"
style="@style/season_name"
>
</TextView>
<TextView
android:id="@+id/winter"
android:text="冬天"
style="@style/season_name"
>
</TextView>
</LinearLayout>
</RelativeLayout>
style.xmlvalues
由于底部的按钮样式一致故将其提取出来写成样式文件进行复用。
<resources>
<style name="season_name">
<item name="android:layout_width">50dp</item>
<item name="android:layout_height">50dp</item>
<item name="android:background">@drawable/buttonstyle</item>
<item name="android:textColor">@color/white</item>
<item name="android:textSize">15sp</item>
<item name="android:gravity">center</item>
<item name="android:layout_margin">10dp</item>
</style>
</resources>
buttonstyle.xmldrawable
底部按钮蓝色圆形背景图的设置
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="oval">
<solid android:color="#2664aa"></solid>
</shape>
功能代码
SeasonActivity.java
使用Java写页面背后的业务逻辑重点在于事件响应机制理解事件、事件源、事件监听器三者区别以及如何书写原理与Java 中AWT或者Swing图形界面类似。
package com.example.app01;
import android.os.Bundle;
import android.view.View;
import android.widget.FrameLayout;
import android.widget.TextView;
import androidx.annotation.Nullable;
import androidx.appcompat.app.AppCompatActivity;
public class SeasonActivity extends AppCompatActivity {
//定义组件类型的变量来接收事件源组件
private TextView Spring;
private TextView Summer;
private TextView Autuom;
private TextView Winter;
private FrameLayout flSeason;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
//指定当前的页面
setContentView(R.layout.text3_season);
initView();
}
//初始化获取组件
private void initView(){
SeasonClick sea = new SeasonClick();
//通过id找到指定组件
Spring=findViewById(R.id.spring);
Summer=findViewById(R.id.summer);
Autuom=findViewById(R.id.autuom);
Winter=findViewById(R.id.winter);
flSeason=findViewById(R.id.frameSeason);
//设置事件监听器
Spring.setOnClickListener(sea);
Summer.setOnClickListener(sea);
Autuom.setOnClickListener(sea);
Winter.setOnClickListener(sea);
}
//内部类实现事件监听
class SeasonClick implements View.OnClickListener{
@Override
public void onClick(View v) {
//类型强转换
TextView txt = (TextView) v;
//获取图片的tag
String tag = txt.getText().toString();
//获取帧式布局中子元素的个数
int count = flSeason.getChildCount();
for (int i = 0; i < count; i++) {
//通过下标获取到组件
View vi = flSeason.getChildAt(i);
//如果图片的tag与所点击的标签按钮一致则图片显示否则设置为隐藏
if(vi.getTag().equals(tag)){
vi.setVisibility(View.VISIBLE);
}else{
vi.setVisibility(View.GONE);
}
}
}
}
}
注意由于安卓默认开机启动项是MainActivity.java文件因此我们要在配置文件中修改为SeasonActivity才能显示本文件如下图所示
其实这里就是在将每一个Activity进行配置后续文章会重点讲解安卓中的Activity。
运行效果
点击下方按钮切换到对应季节的图片
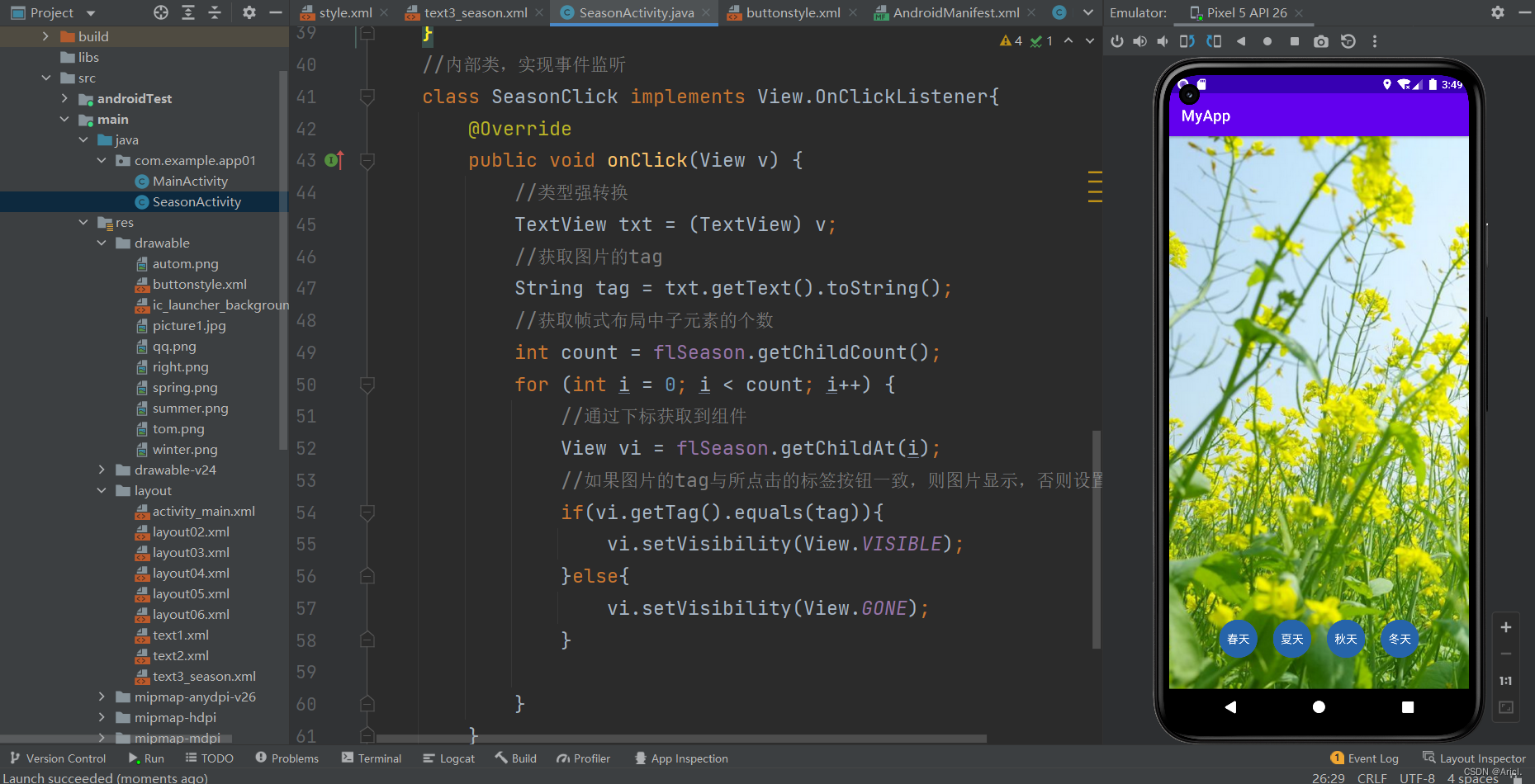
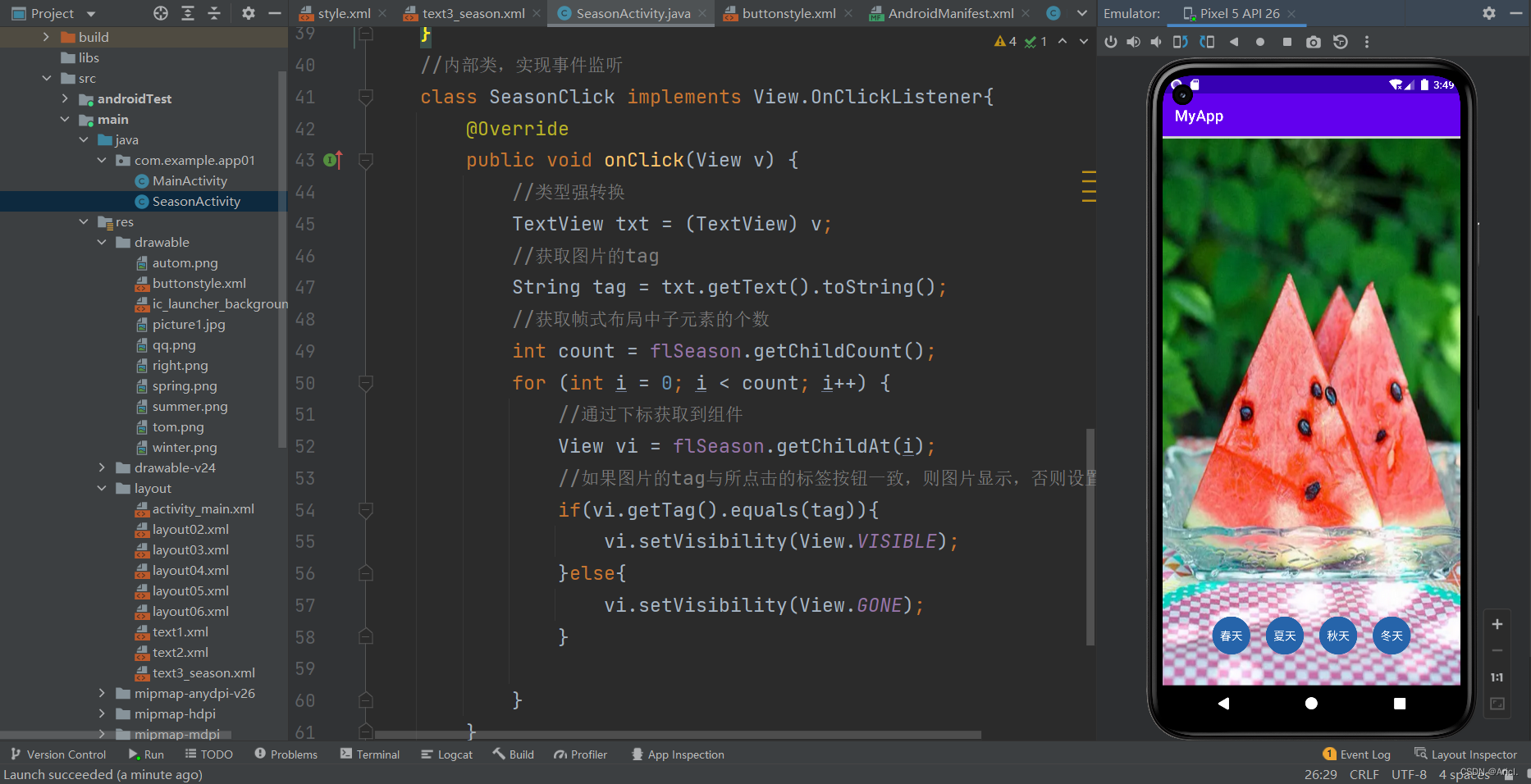
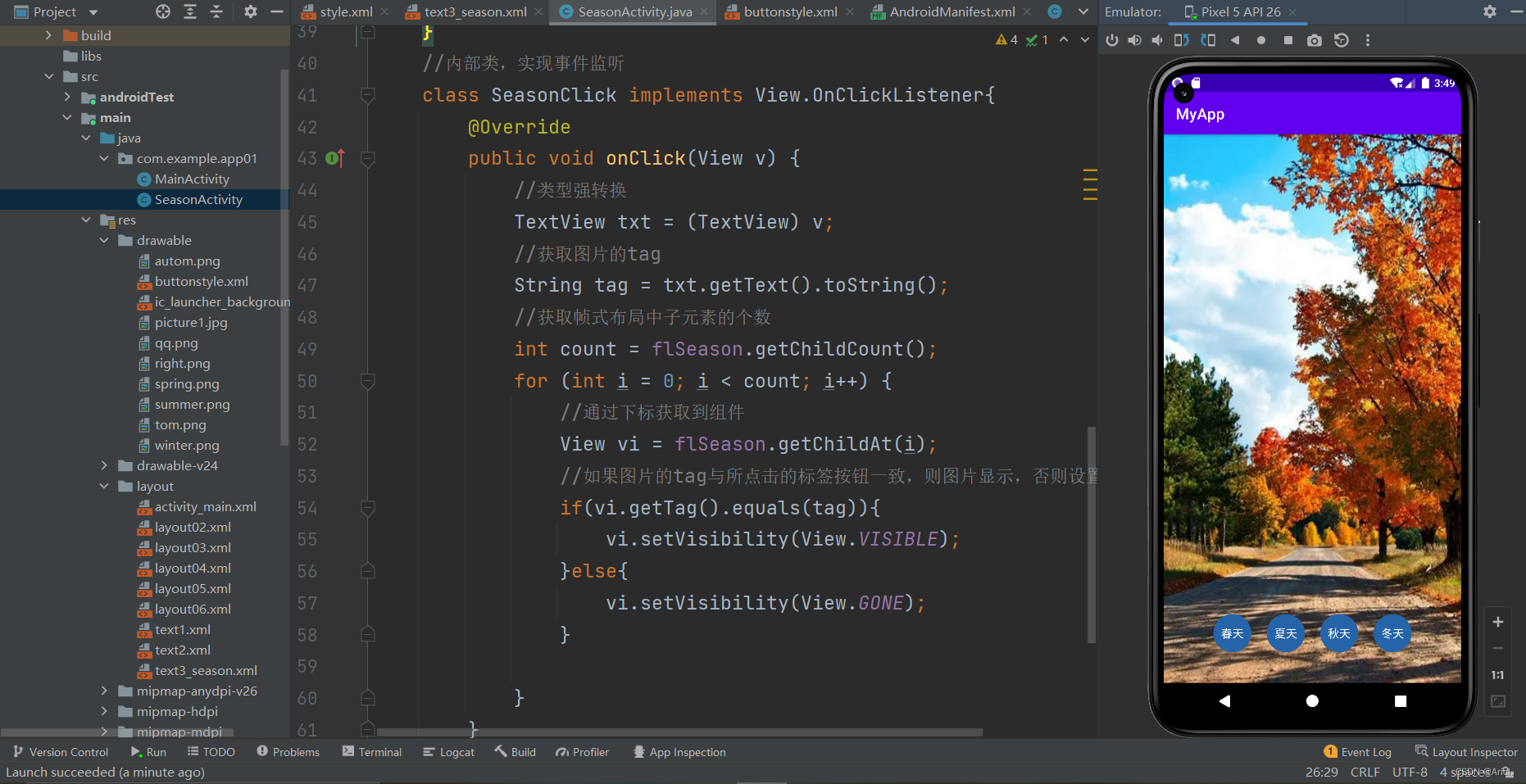
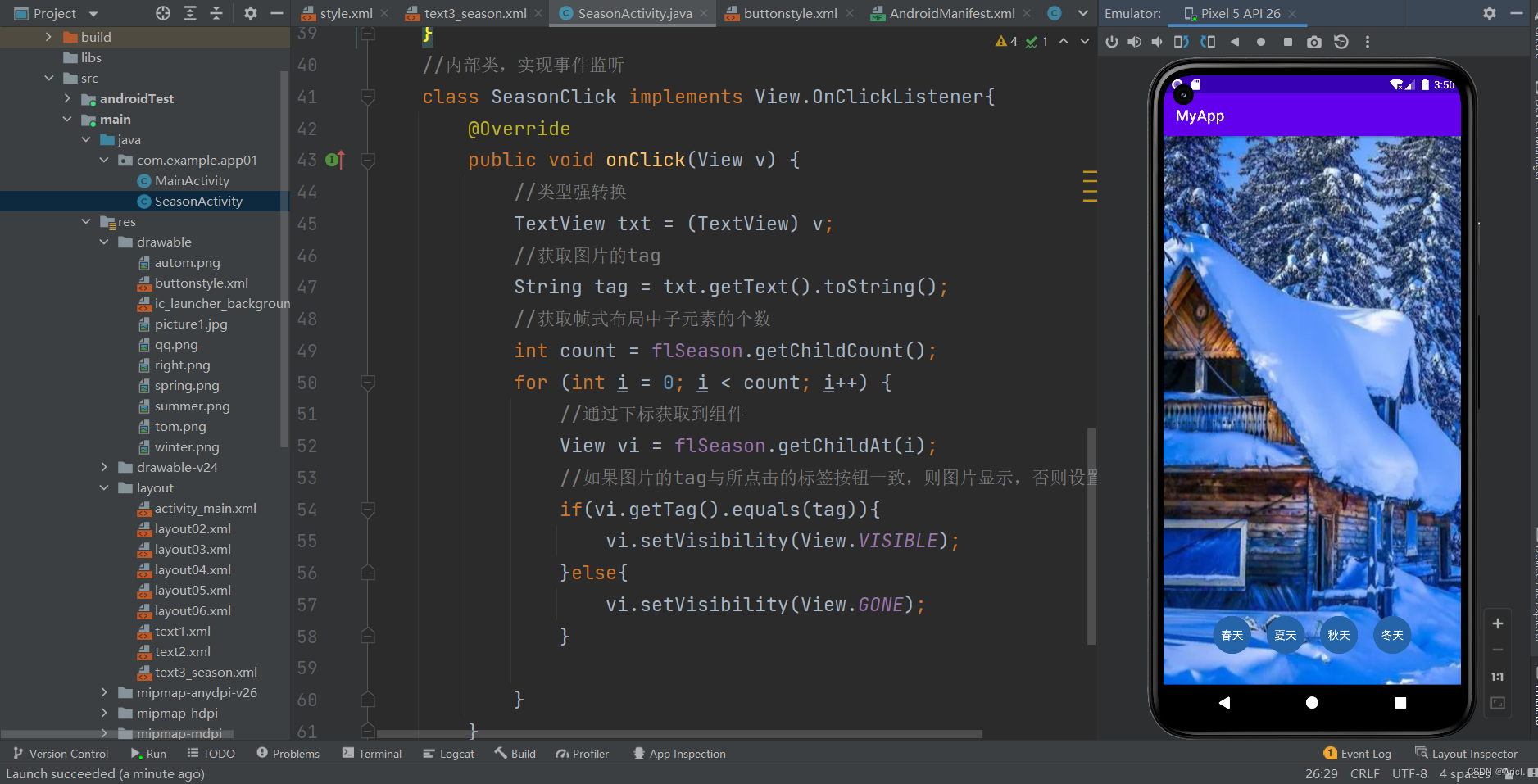
END.